3D Physics Simulation¶
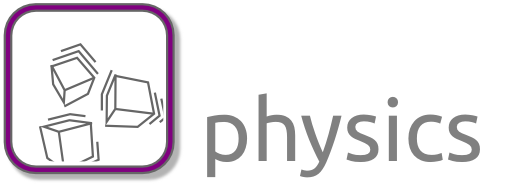
The ICLPhysics package provides a powerful wrapper layer around the most common aspects of the versatile Bullet Physics engine (http://bulletphysics.org). The implemented wrapper layout features soft and rigid body dynamics and collisions as well as constraints and motor types. Its seamless integration with ICLGeom’s rendering API allows for super fast rapid prototyping.
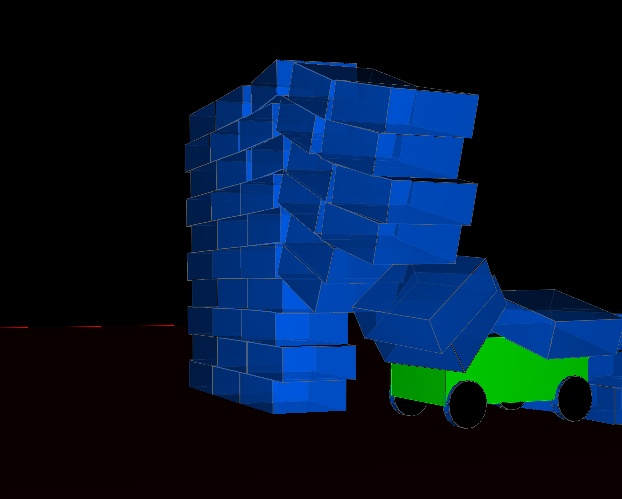
Table of Contents¶
PhysicsScene¶
The PhysicsScene extends the PhysicsWorld and the Scene. It provides global settings like gravity and collision groups, as well as utility functions to test rays as well as objects for collision with the objects within the PhysicsWorld. PhysicsObject and Constraint can be added and behave just like SceneObjects. The simulation can be stepped through my simply calling the step function. For further information on how to fine-tune the step parameters visit http://bulletphysics.org/mediawiki-1.5.8/index.php/Stepping_The_World.
RigidObject¶
The physics::RigidObject class is one of the two basic types of objects in the physics scene. The subclasses like RigidBoxObject or RigidCylinderObject allow for quick scene building with primitive shapes. For more complex shapes RigidConvexHullObject can be used to create a RigidObject from a vector of points. The RigidCompoundObject allows to create concave objects by combining other RigidObjects. RigidObject have parameters like weight, friction, or restitution which allows for modeling different behaviour when objects collide.
Here is an example for a simple scene with some objects:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <ICLGeom/Geom.h>
#include <ICLQt/Common.h>
#include <ICLUtils/FPSLimiter.h>
#include <ICLPhysics/PhysicsScene.h>
#include <ICLPhysics/RigidBoxObject.h>
#include <ICLPhysics/RigidCylinderObject.h>
#include <ICLPhysics/RigidSphereObject.h>
#include <ICLPhysics/PhysicsMouseHandler.h>
using namespace geom;
using namespace physics;
GUI gui;
FPSLimiter fps(60);
Camera cam(Vec(2000,0,500,1), Vec(-1,0,0,1), Vec(0,0,-1,1));
//defining the position, dimensions and weight of the objects in the scene
RigidBoxObject box(0,0,500, 100, 100, 100, 0.1);
RigidCylinderObject cylinder(0,-20,700.0, 100, 100 , 0.1);
RigidSphereObject sphere(-200,0,90,100,0.1);
//a weight of zero makes objects static
RigidBoxObject table(0,0,-200.0, 10000, 10000, 200, 0);
PhysicsScene scene;
PhysicsMouseHandler handler(0,&scene);
void init(){
gui << Draw3D().handle("draw") << Show();
scene.addCamera(cam);
table.setColor(Primitive::all,geom_red());
//restition defines how bouncy an object is
cylinder.setRestitution(0.5f);
sphere.setRestitution(0.5f);
box.setRestitution(0.5f);
table.setRestitution(0.9f);
//friction describes the damping behaviour when two objects slide against each other
box.setFriction(0.5f);
cylinder.setFriction(0.5f);
sphere.setFriction(0.5f);
table.setFriction(0.5f);
//rolling frction describes the angular damping behaviour when one object rolls over another
cylinder.setRollingFriction(0.2f);
sphere.setRollingFriction(0.1f);
table.setRollingFriction(0.2f);
box.setRollingFriction(0.1f);
scene.addObject(&cylinder);
scene.addObject(&sphere);
scene.addObject(&box);
scene.addObject(&table);
gui["draw"].install(&handler);
//link the visualization
gui["draw"].link(scene.getGLCallback(0));
}
void run()
{
scene.step();
gui["draw"].render();
fps.wait();
}
int main(int n, char**ppc){
return ICLApplication(n,ppc,"",init,run).exec();
}
|
SoftObject¶
The SoftObject is the wrapper class for the bullet softbodies. Softbodies enable simulation of pliable bodies like cloth and rubber at a greater cost of performance.
Constraints¶
Constraints allow the simulation of behaviour like hinges, ball sockets or rails. These constraints are all implemented based on the SixDofConstraint which allows for generic constraints. The constraint works by describing 2 transformations matrices which represnt a positoins and orientation (in bullet these are called frames) relativ to two objects. When all six degrees of freedem(3 translation and 3 rotation degrees) are locked the constraint will try move these frames on top of each other. By unlocking certain degrees free traversal along that degree can be allowed.
The following code will create an axis with the length of 2 units between the objects A and B, where both objects can turn at most rotate 180° away from each other:
1 2 3 4 5 6 7 8 9 10 11 | SixDofConstraint *c = new SixDOFConstraint(objectA,objectB,
Mat(1,0,0,0,
0,1,0,0,
0,0,1,-1,
0,0,0,1),
Mat(1,0,0,0,
0,1,0,0,
0,0,1,1,
0,0,0,1));
c->setAngularLowerLimit(Vec(0,0,0));
c->setAngularUpperLimit(Vec(0,0,M_PI));
|
For more instructions on how constraints work check out the physics-constraint and the physics-car demo or visit http://bulletphysics.org/mediawiki-1.5.8/index.php/Constraints.
Dimensions¶
When constructing scenes it is always important to keep an eye on proportions. The physics work best when objects have a size of about 1. This is where the two global variables ICL_UNIT_TO_METER and METER_TO_BULLET_UNIT come into play. The default unit in ICL is the millimeter therefore ICL_UNIT_TO_METER is 0.001. When working with objects that are about 10 cm in size METER_TO_BULLET_UNIT should be set to 0.1. Since objects in the ICL usually are about this big 0.1 is the default value. One of the limitations of the bullet physics engine is the interaction between very small/light and big/heavy objects. In these cases rounding errors can result in unexpected behaviour.